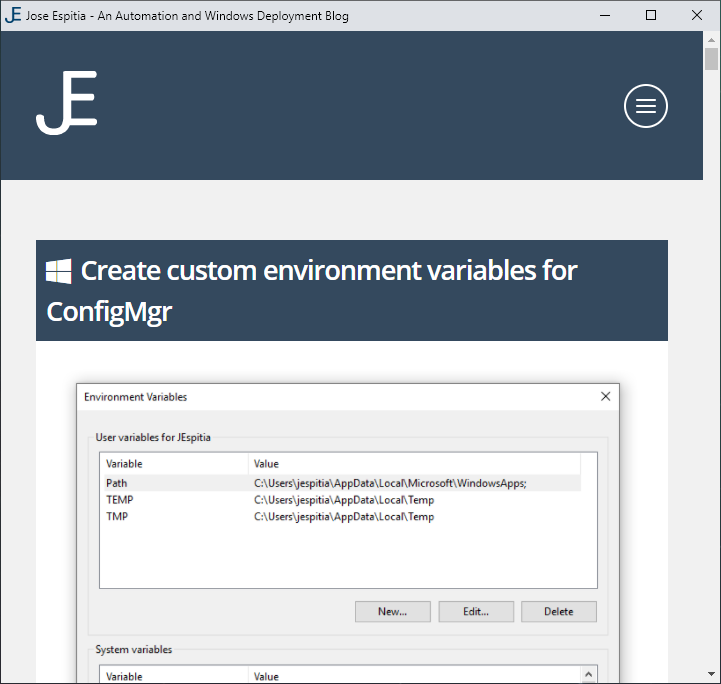
Use the New-ChromeWebApp Powershell function to automatically create Google Chrome web application shortcuts.
Example: Create a web application shortcut for JoseEspitia.com in the Start Menu
New-ChromeWebApp -Location "C:\ProgramData\Microsoft\Windows\Start Menu\Programs\Jose Espitia.lnk" -URL https://www.JoseEspitia.com -Icon https://www.JoseEspitia.com/favicon.ico
Function:
Function New-ChromeWebApp {
<#
.SYNOPSIS Create Chrome Web Applications
.PARAMETER Location - Specify location for the shortcut
.PARAMETER URL - Specify the web apps URL
.PARAMETER Icon - Specify location for shortcut icon
.EXAMPLE - Create JoseEspitia.com web app in All Users start menu
New-ChromeWebApp -Location "C:\ProgramData\Microsoft\Windows\Start Menu\Programs\Jose Espitia.lnk" -URL https://www.JoseEspitia.com -Icon https://www.JoseEspitia.com/favicon.ico
#>
param (
[parameter(Mandatory=$True)]
[string]$Location,
[parameter(Mandatory=$True)]
[string]$URL,
[parameter(Mandatory=$True)]
[string]$Icon
)
# Get Chrome.exe path
$ChromeOpenCommand = (Get-ItemProperty Registry::HKCR\ChromeHTML\shell\open\command)."(Default)"
$ChromeDefaultPath = $ChromeOpenCommand.Substring(0, $ChromeOpenCommand.IndexOf(' --'))
# Create web app shortcut
$Shortcut = (New-Object -ComObject WScript.Shell).CreateShortcut("$Location")
$Shortcut.TargetPath = "$ChromeDefaultPath"
$Shortcut.Arguments = "--app=$URL"
$Shortcut.WorkingDirectory = "C:\Program Files\Google\Chrome\Application"
$Shortcut.IconLocation = "$Icon"
$Shortcut.Save()
}