This universal script will automate your BIOS updates in MDT. In order for this script to work, you will need to configure your MDT deployment share with the following folder structure:
DeploymentShare$\Scripts\Custom\BIOS
Inside of the BIOS folder, you will need a folder for each model that you are supporting in your deployment. The folder names must match the model name that MDT queries with ZTIGather.
You can run wmic computersystem get model to get this value.
Folder Structure Example:
DeploymentShare$\Scripts\Custom\BIOS\10HY002AUS
DeploymentShare$\Scripts\Custom\BIOS\HP EliteBook 8560w
Inside of these folders, you will need to place all the files needed to install your BIOS update. You will also need to create custom files needed to silently install and determine the latest BIOS version.
1st File: BIOS.txt
In this txt file, you will place the BIOS version of the update. This is used to compare the BIOS version installed on the machine and the latest update version.
Example: FBKTCCAUS
2nd File: UpgradeBIOS.cmd
In this file you will add all the commands needed to silently install your BIOS update.
Example:
REM Setting Current Directory
cd "%~dp0"
WINUPTP.exe -s
Once you have the the folder structure completed, you will want to add a Reboot task to your Task Sequence. With this task, you will need to add an if statement with the following configuration:
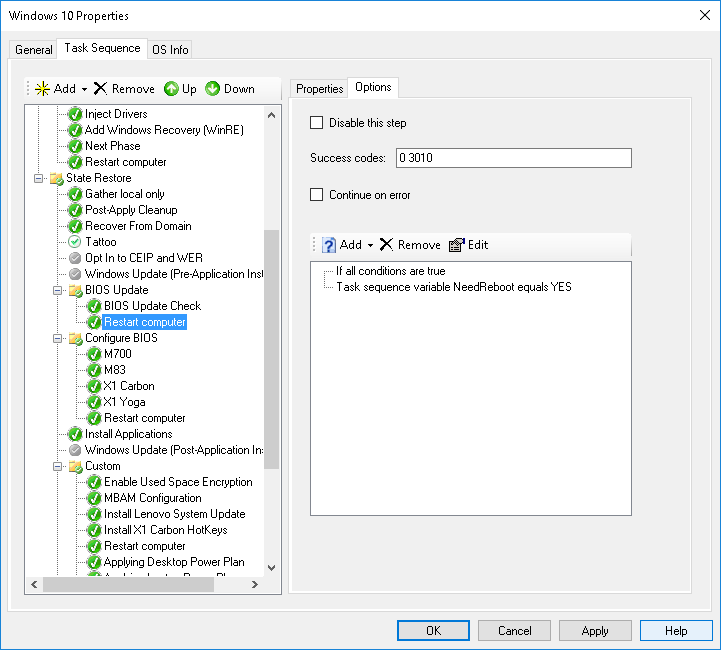
And now for the actual Powershell script!
# Load MDT Task Sequence Environment and Logs
$TSenv = New-Object -COMObject Microsoft.SMS.TSEnvironment
$logPath = $tsenv.Value("LogPath")
$logFile = "$logPath\BIOS_Update.log"
# Start the logging
Write-Output "Logging to $logFile." > $logFile
# Collect data
Write-Output "Collecting Data" >> $logFile
$ScriptRoot = (Get-location).Path
$Model = $TSenv.Value("Model")
$CompBiosVersion = (Get-WmiObject WIN32_BIOS).SMBIOSBIOSVersion
$CurrentBiosVersion = Get-Content "$ScriptRoot\$Model\BIOS.txt"
$Installer = "UpgradeBIOS.cmd"
try {
Test-Path $CurrentBiosVersion -ErrorAction Stop
}
catch {
Write-Output "BIOS.txt does not exist!" >> $logFile
}
Write-Output "Copying $ScriptRoot\$Model to C:\Temp\$Model" >> $logFile
Copy-Item "$ScriptRoot\$Model" "C:\Temp\$Model" -Force -Recurse
# Checking for BIOS update
if($CompBiosVersion.replace(' ' , '') -eq $CurrentBiosVersion.replace(' ' , '')) {
Write-Output "BIOS is up to date." >> $logFile
Exit
}
else {
Write-Output "Updating BIOS $CompBiosVersion to $CurrentBiosVersion." >> $logFile
Start-Process "cmd.exe" "/c C:\Temp\$Model\$Installer" -Wait
$tsenv.Value("NeedReboot") = "YES"
Write-Output "Update has been completed successfully." >> $logFile
Exit
}